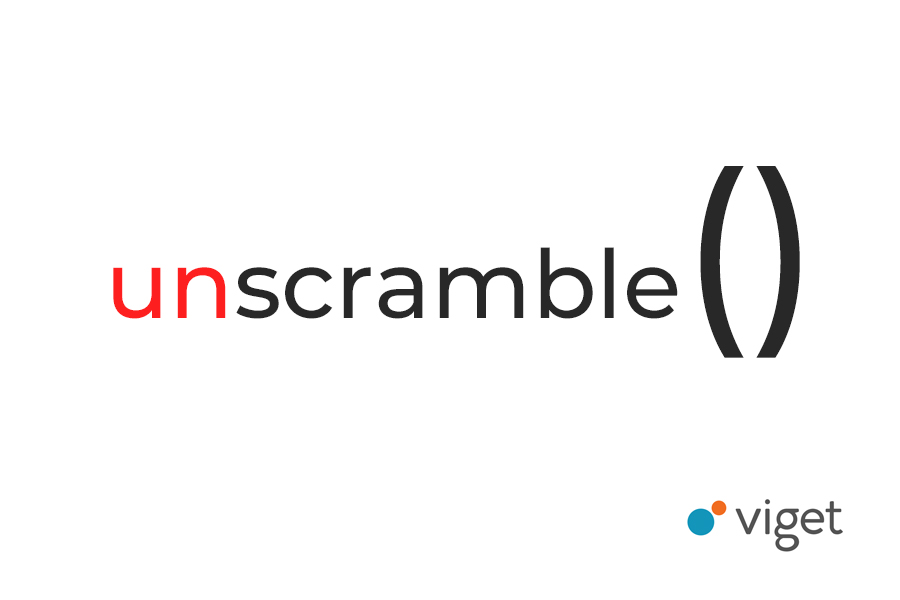
Viget Labs Unscramble Challenge
The Challenge
Viget, which is one of the agencies I follow and have learned from, recently put out a challenge lab for people to work through. The challenge was simple enough. Using whatever language you wanted you were to write a solution for a program that unscrambles a scrambled word. As I looked through the solutions, I was shocked that no one had written a solution in PHP yet. So I did.
Below is a video showing the way I went about doing it. If you would like to see/copy any of my code, then you can do that below the video or you can go to git the repo on GitHub.
The Work
The Solution
Below is the function to be able to unscramble any word in the English dictionary. Keep in mind that if you use a word that shares the same letters and length with another word, then the first one that comes alphabetically will be used. For instance, “nac” will be returned as “anc” rather than “can.”
Enjoy.
<?php
//Take scrambled into and output a single unscrambled word
function unscramble($input){
$output = '';
//Collect an array of English words
$words = json_decode(file_get_contents('https://raw.githubusercontent.com/dwyl/english-words/master/words_dictionary.json'), true);
//Split our input into letters and sort alphaabetically
$input_array = str_split($input);
sort($input_array);
//Loop through the array of words and split each word alphaabetically
foreach($words as $word => $number){
$word_array = str_split($word);
sort($word_array);
if($input_array === $word_array){
$output = $word;
break;
}
}
return $output;
}
?>